Build Angular authentication with Logto
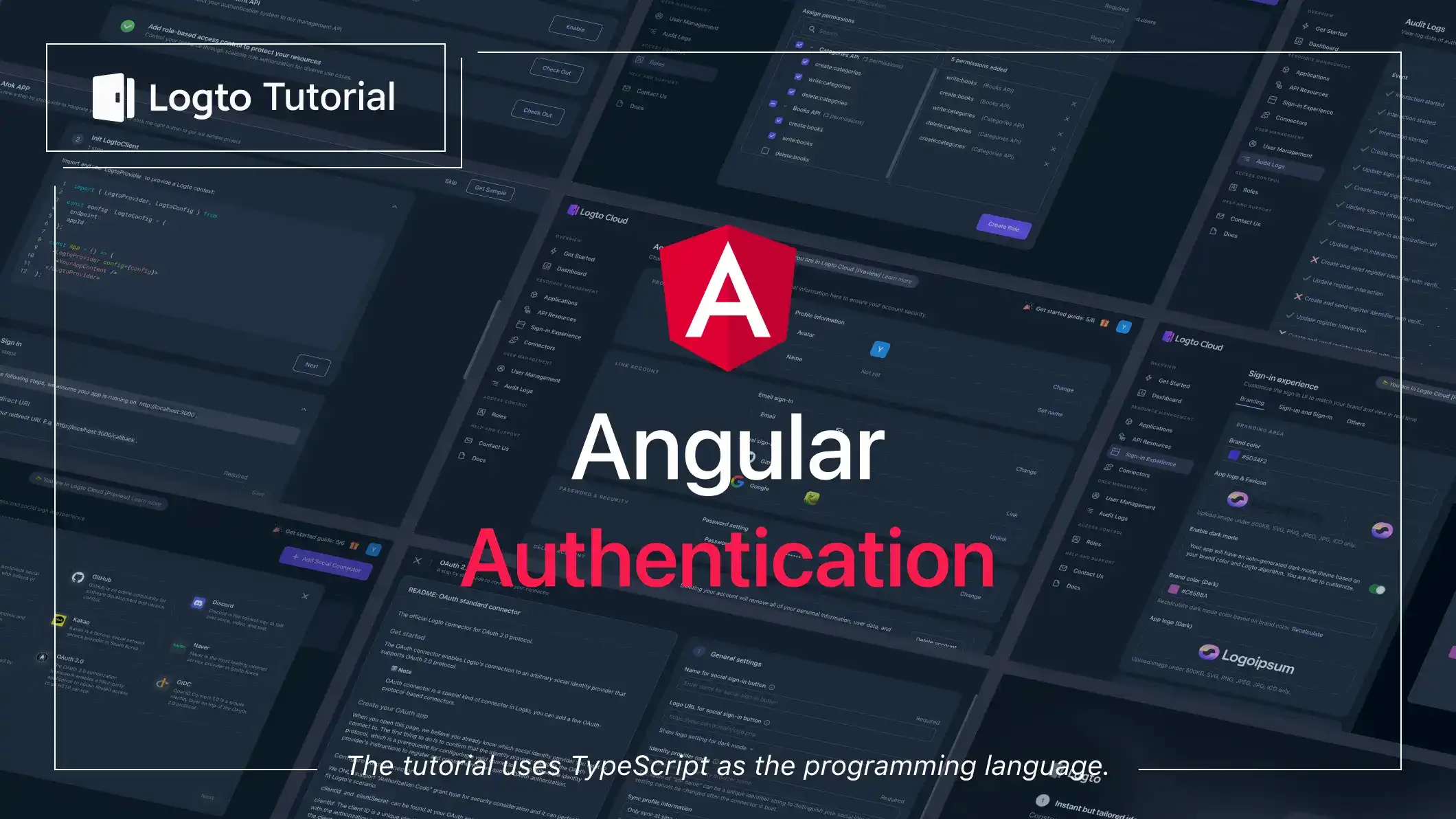
Get started
Introduction
- Logto is an open-source Auth0 alternative for building identity infrastructures. It supports various sign-in methods, including username, email, phone number, and popular social sign-ins like Google and GitHub.
- Angular is a web development framework created and maintained by Google.
In this tutorial, we will show you how to build a user authentication flow with Angular by integrating Logto SDK. The tutorial uses TypeScript as the programming language.
Prerequisites
Before you begin, ensure you have the following:
- A Logto account. If you don't have one, you can sign up for free.
- An Angular development environment and a project.
Create a Logto application
To get started, create a Logto application with the "Single page app" type. Follow these steps to create a Logto application:
- Sign in to the Logto Console.
- In the left navigation bar, click on Applications.
- Click on Create application.
- In the opened page, find the "Single page app" section and locate the "Angular" card.
- Click on Start building, and input the name of your application.
- Click on Create.
Then you should see an interactive tutorial that guides you through the process of integrating Logto SDK with your Angular application. The following content can be a reference for future use.
Integrate Logto to your project
Installation
Install Logto JS core SDK and Angular OIDC client library:
# or pnpm, yarn, etc.
npm i @logto/js angular-auth-oidc-client
Configure application
In your Angular project, add the auth provider your app.config.ts
:
import { UserScope, buildAngularAuthConfig } from '@logto/js';
import { provideAuth } from 'angular-auth-oidc-client';
export const appConfig: ApplicationConfig = {
providers: [
provideHttpClient(withFetch()),
provideAuth({
config: buildAngularAuthConfig({
endpoint: '<your-logto-endpoint>',
appId: '<your-app-id>',
scopes: [UserScope.Email], // Add more scopes if needed
redirectUri: 'http://localhost:3000/callback',
postLogoutRedirectUri: 'http://localhost:3000/',
}),
}),
// ...other providers
],
};
Implement sign-in and sign-out
http://localhost:3000/
.Configure redirect URIs
Switch to the application details page of Logto Console. Add a Redirect URI http://localhost:3000/callback
.
Redirect URI is an OAuth 2.0 concept which implies the location should redirect after authentication.
Similarly, add http://localhost:3000/
to the "Post sign-out redirect URI" section.
Post Sign-out Redirect URI is an OAuth 2.0 concept which implies the location should redirect after signing out.
Then click "Save" to save the changes.
In the component where you want to implement sign-in and sign-out (for example, app.component.ts
), inject the OidcSecurityService
and use it to sign in and sign out.
import { OidcSecurityService } from 'angular-auth-oidc-client';
export class AppComponent implements OnInit {
constructor(public oidcSecurityService: OidcSecurityService) {}
signIn() {
this.oidcSecurityService.authorize();
}
signOut() {
this.oidcSecurityService.logoff().subscribe((result) => {
console.log('app sign-out', result);
});
}
}
Then, in the template, add buttons to sign in and sign out:
<button (click)="signIn()">Sign in</button>
<br />
<button (click)="signOut()">Sign out</button>
Subscribe to authentication state and display user information
The OidcSecurityService
provides a convenient way to subscribe to the authentication state:
import { OidcSecurityService } from 'angular-auth-oidc-client';
import type { UserInfoResponse } from '@logto/js';
export class AppComponent implements OnInit {
isAuthenticated = false;
userData?: UserInfoResponse;
idToken?: string;
accessToken?: string;
constructor(public oidcSecurityService: OidcSecurityService) {}
ngOnInit() {
this.oidcSecurityService
.checkAuth()
.subscribe(({ isAuthenticated, userData, idToken, accessToken }) => {
console.log('app authenticated', isAuthenticated, userData);
this.isAuthenticated = isAuthenticated;
this.userData = userData;
this.idToken = idToken;
this.accessToken = accessToken;
});
}
// ...other methods
}
And use it in the template:
<button *ngIf="!isAuthenticated" (click)="signIn()">Sign in</button>
<ng-container *ngIf="isAuthenticated">
<pre>{{ userData | json }}</pre>
<p>Access token: {{ accessToken }}</p>
<!-- ... -->
<button (click)="signOut()">Sign out</button>
</ng-container>
Checkpoint: Run the application
Now you can run the application and try to sign-in/sign-out with Logto:
- Open the application in your browser, you should see the "Sign in" button.
- Click the "Sign in" button, and you should be redirected to the Logto sign-in page.
- After you have signed in, you should be redirected back to the application, and you should see the user data and the "Sign out" button.
- Click the "Sign out" button, and you should be redirected to the Logto sign-out page, and then redirected back to the application with an unsigned-in state.
If you encounter any issues during the integration, please don't hesitate to join our Discord server to chat with the community and the Logto team!