Client assertion in OAuth 2.0 client authentication
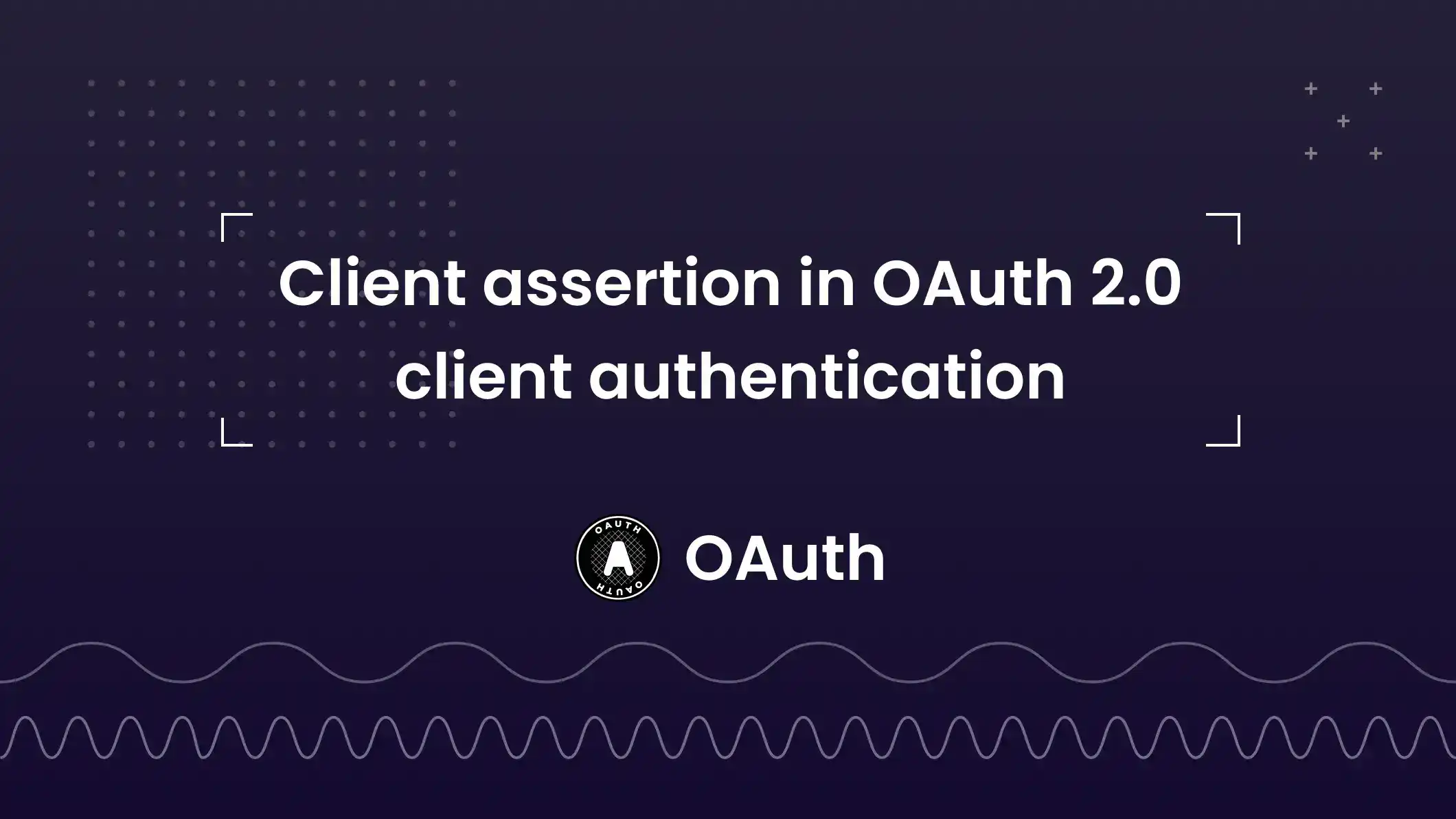
As the demand for web and application security grows, OAuth 2.0 has become a key technology for authorizing access to resources. Its security and efficiency have garnered widespread attention. This article delves into the difference between traditional client ID and secret authentication and client assertions, introducing the use of client assertions in OAuth 2.0 client authentication.
Introduction to client authentication
In OAuth 2.0, a "client" refers to an application requesting access to a resource server. Client authentication is the process by which the authorization server verifies the identity of the requesting client.
Let's explain client authentication behavior with two common OAuth authentication flows:
- Authorization code flow: Here, the client first needs user authorization (usually clicking a consent button on a user consent page) to obtain an authorization code. Then, the client uses this code and credentials (usually a
client_id
andclient_secret
) for authentication and requests an access token from the authorization server. - Client credentials flow: In this flow, the client uses its credentials (usually a
client_id
andclient_secret
) to request an access token directly from the authorization server without a user authorization step.
Client authentication with client ID and client secret
The most common method for client authentication is using a client ID and client secret.
Take the client credentials flow as an example. The client sends a POST request to the authorization server's token endpoint with related client credentials:
const fetch = require('node-fetch');
const clientId = 'Your client ID';
const clientSecret = 'Your client secret';
const tokenEndpoint = 'https://authorization-server.com/token';
const body = new URLSearchParams({
grant_type: 'client_credentials',
client_id: clientId,
client_secret: clientSecret,
});
fetch(tokenEndpoint, {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: body,
})
.then((response) => response.json())
.then((accessToken) => console.log(accessToken))
.catch((error) => console.error('Error:', error));
This straightforward method is easy for developers to deploy quickly and is supported by nearly all OAuth service providers. However, it has limitations in scenarios with high security and flexibility demands:
- The client secret is transmitted in requests, making it vulnerable to interception over insecure networks.
- The secret can be easily accessed by unrelated services within an internal network where services communicate with each other without TLS.
- The fixed combination of client ID and secret is susceptible to replay attacks.
- Relying solely on client ID and secret for authentication limits the mechanism's flexibility and prevents carrying more client metadata or custom information.
Introduction to client assertion
In OAuth 2.0, client assertion offers a more efficient and secure method for client authentication. Compared to the traditional client ID and secret, client assertion uses JSON Web Tokens (JWT) to enhance security and flexibility, making the authentication process more reliable and informative.
JWTs are compact and self-contained, securely transmitting information between parties as JSON objects. A JWT contains claims about an entity (usually the user) and other data, including:
- iss (Issuer): The claimant, usually the client ID, indicating who created the JWT.
- sub (Subject): Also typically the client ID, indicating the subject of the JWT.
- aud (Audience): Referring to the URL of the authorization server's token endpoint, showing who the JWT is intended for.
- exp (Expiration Time): The expiry time after which the JWT is no longer accepted.
- iat (Issued At): The issuance time, marking when the JWT was created.
- jti (JWT ID): A unique identifier for the JWT, mainly for preventing the JWT from being replayed.
This combination of information provides unmatched security over traditional client secret authentication, adding flexibility and control capabilities.
Using client assertion for client authentication
Let's demonstrate client authentication using client assertion with the OAuth 2.0 client credentials flow, mainly applied when the client requests an access token on its behalf, without direct user involvement.
When authenticating with a client assertion in OAuth 2.0, the client_assertion_type
must be urn:ietf:params:oauth:client-assertion-type:jwt-bearer
, and the client_assertion
parameter carries the client's JWT assertion. Here's a Node.js code example for generating a JWT assertion for client authentication:
const jwt = require('jsonwebtoken');
const fetch = require('node-fetch');
const clientId = 'Your client ID';
const clientSecret = 'Your client secret';
const tokenEndpoint = '<https://authorization-server.com/token>';
const clientAssertion = jwt.sign(
{
iss: clientId,
sub: clientId,
aud: tokenEndpoint,
exp: Math.floor(Date.now() / 1000) + 60 * 60, // Expires in 1 hour
iat: Math.floor(Date.now() / 1000),
},
clientSecret,
{
// Algorithm specified for signing the token (supported by the authentication server).
algorithm: 'HS256',
}
);
const body = new URLSearchParams({
grant_type: 'client_credentials',
client_assertion_type: 'urn:ietf:params:oauth:client-assertion-type:jwt-bearer',
client_assertion: clientAssertion,
client_id: clientId,
});
fetch(tokenEndpoint, {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: body,
})
.then((response) => response.json())
.then((accessToken) => console.log(accessToken))
.catch((error) => console.error('Error:', error));
Ensure the client secret's security and take appropriate measures to prevent its disclosure.
Choosing client authentication method
As discussed, each authentication method has its advantages and applicable scenarios. When integrating OAuth 2.0 services, choose the most suitable option based on specific needs.
Client assertion, with their advanced encryption technologies, provide data protection and support complex authentication scenarios, allowing for easy future expansion. However, due to their complexity and the need for a deep understanding of JWT and its encryption mechanisms, simpler client ID and secret authentication might be more appropriate for teams with limited resources or those looking for quick deployment.
Summary
This article discussed the application of client assertions in OAuth 2.0 client authentication, comparing it with traditional client ID and secret authentication methods. Client assertion offers enhanced security and flexibility for complex security needs but also imply higher implementation complexity. In practice, choose the most suitable option based on specific requirements and technical expertise to meet business development needs.